Hello, Coders!! In this article, we will learn how to create a tip calculator in JavaScript. This project is ideal for beginners. If you are learning javascript, this project can help you understand the concepts more clearly.
Tip Calculator is basically an web application that will help to do Tip Calculation.
How To Make Simple Tip Calculator Using Javascript?
For instance, suppose you and a friend went to a hotel for dinner and the bill was $10. Because you enjoy the hotel’s food and services, you and your friend decided to leave a tip for the waiter.In such a case, there is no other option than to use this type of project to calculate how much money or dollars each person must pay.
You will have to work much harder if you do this manually. Because the goal of programming is to make our daily tasks easier, this type of project will greatly simplify your calculation. You will only need to enter information here, and the result will be displayed automatically.
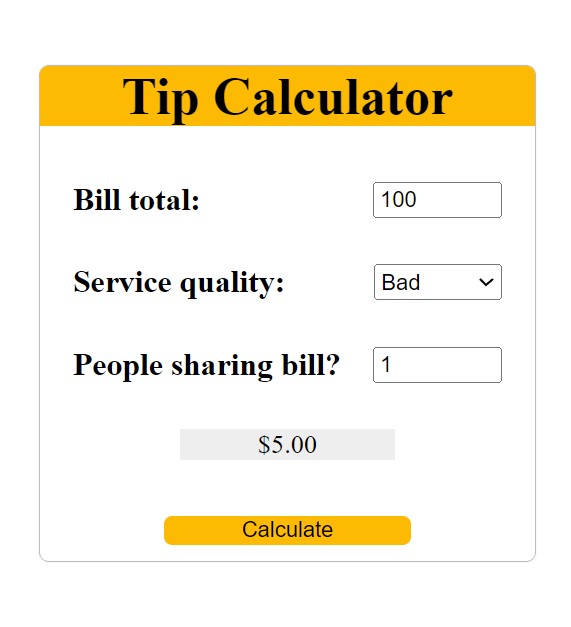
I hope you have a general idea of what the project entails. In our article, we will go over this project step by step.
How To Make A Simple Tip Calculator Using Javascript
We have created three input areas in this tip calculator, two of which are of the number type, in which you will fill in the amount of the bill and the number of people sharing the bill, and there is also a drop-down list from which you will select how much you like the waiter’s service, such as “Bad,” “Good,” and “Excellent”.
Step1: Basic Structure of Tip Calculator
The Simple Tip Calculator’s basic structure was created using the HTML and CSS code below. I’ve have design a container here to show you the basic structure in which all of the information can be found.
We added the style to our tip form by using the ID selector. We’ve added a box-shadow and a border-radius. The background is white, and the display is set to “flex,” with the flex oriented column-wise. The width and height are both set to “300px.”
<div class="container"> <form action="index.html" method="post" id="tipForm"> </form> </div>
* { box-sizing: border-box; } body { /* background: #222; */ background-repeat: no-repeat; height: 100vh; margin: 0; } /* ------------- FORM STYLES ----------------*/ .container { } #tipForm { border-radius: 5px; box-shadow: 0 0 1px; position: absolute; left: 40%; top: 20%; background: #fff; display: flex; flex-direction: column; height: 300px; width: 300px; padding-bottom: 10px; }
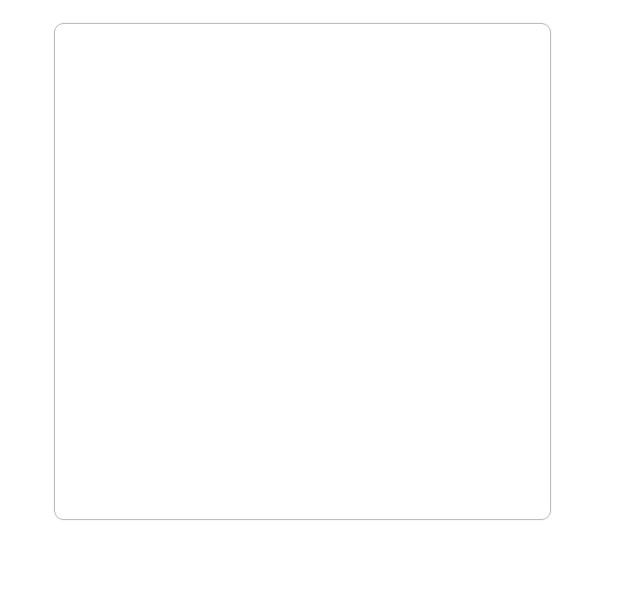
Step2: Adding a Basic Heading to our Tip Calculator
Now using the H1 tag we have added the heading to our Tip calculator . This heading is primarily intended to enhance the beauty. The background colour of this heading is yellow and the text colour is black.
<h1>Tip Calculator</h1>
h1 { border-radius: 5px 5px 0 0; background-color: #fcba03; margin: 0; text-align: center; padding: 0 20px; }

Step3: Adding inputs to our Tip Calculator
Now, we’ll use the div tag to create a container for our input, and within it, we’ll add a label for “Bill Amount,” followed by the bill amount using the input type “number.”
Similarly, for our second section on dropdown select menus, we will use the select tag to add the option.
100+ Front-end Projects for Web developers (Source Code)
Then, using the same method as for creating the bill amount input, we will create the input for our number of people sharing, followed by a blank div that will display the result and a button created with a div tag.
Using css we will add style to our input box and button so that the look more appealing.
<label for="bill_amount">Bill total:</label> <input type="number" name="bill_amount" id="bill" min="0" placeholder="$0"> </div> <div class="flex"> <label for="service_quality">Service quality:</label> <select name="service_quality" id="service" form="tipForm"> <option value="0.05">Bad</option> <option value="0.10">Good</option> <option value="0.20">Excellent</option> </select> </div> <div class="flex"> <label for="split_bill">People sharing bill?</label> <input type="number" name="split_bill" id="split" min="1" placeholder="0"> </div> <div id="result">$--</div> </div> <div class="flex-btn"> <button type="button" onclick="calculateTip()" id="calc-btn">Calculate</button> </div>
We’ll style our input box with the input class. We set the display to “flex” and the flex-direction to “column.” We also added a 20px margin to our inputs.
Using the label tag, we will specify the font-size as “1.2 rem” and the font-weight as “bold.”
We’ll add some styling to our button now by using the button tag. With a golden yellow background, the border was set to “none” and the border radius to “5 px.” The display is set to inline block, and the text is centered.
.inputs { display: flex; flex: 1; flex-direction: column; justify-content: space-around; margin: 20px; } label { font-size: 1.2em; font-weight: bold; } #result { align-self: center; background-color: #eee; text-align: center; width: 50%; } button { border: none; border-radius: 5px; background: #fcba03; display: inline-block; text-align: center; width: 50%; } button:hover { background: #c79200; } #split, #bill { width: 30%; } .flex { display: flex; justify-content: space-between; } .flex-btn { display: flex; justify-content: space-around; }
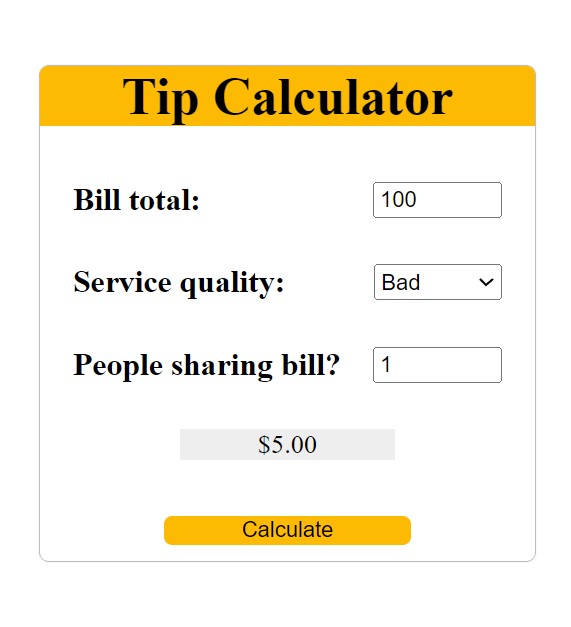
Step3: Adding Javascript to our Tip Calculator
We’ve already established the fundamental structures. It is now time to use JavaScript to complete this project (Simple Tip Calculator in JavaScript). As I previously stated, you require some JavaScript concepts.
First and foremost, we will create an empty array. Now we’ll create a calculatorTip function, a variable called “tip,” and use the getData() function to retrieve data.
We’ll perform a calculation using the data from the array. The data at index 0 will be multiplied by the data at index 1, and then divided by the data at index 2.
ADVERTISEMENT
We now add a condition: if the tip is NaN, the tip value will be displayed as a dollar sign with blanks.
ADVERTISEMENT
Unless otherwise specified, the tip value will be displayed to the nearest two decimal points.
ADVERTISEMENT
We’ve written a getData function and are using it with the document. We will get the value from the html inputs using getElementById. A conditional statement was also added: if the bill’s value is equal to or less than zero, an alert will appear prompting you to enter a valid bill amount. If there is only one person and no splitting, the user must enter one for himself in the input box.
ADVERTISEMENT
var data = []; function calculateTip() { var tip; getData(); tip = (data[0] * data[1]) / data[2]; if (isNaN(tip)) { document.getElementById('result').textContent = '$--'; } else if (tip === Infinity) { document.getElementById('result').textContent = '$--'; } else { document.getElementById('result').textContent = '$' + tip.toFixed(2); } } function getData() { data[0] = document.getElementById('bill').value; data[1] = document.getElementById('service').value; data[2] = document.getElementById('split').value; if (data[0] <= 0) { alert('Please enter a bill amount.'); } else if (data[2] < 1) { alert('Please enter 1 for yourself or more if you are splitting the bill.'); } }
We now have the functionality to our age calculator . Let’s watch a quick video to see how it works.
ADVERTISEMENT
Output:
Codepen Preview Of Tip Calculator using HTML , CSS & Javascript
Now We have Successfully added Tip Calculator using HTML , CSS & Javascript. You can use this project directly by copying into your IDE. WE hope you understood the project , If you any doubt feel free to comment!!
Age Calculator Using Javascript
If you find out this Blog helpful, then make sure to search code with random on google for Front End Projects with Source codes and make sure to Follow the Code with Random Instagram page.