Introduction
Welcome Back to our New Tutorial !! Today we’ll be creating the Intreactive dynamic Quiz App Purely made with ReactJS. In this quiz app there willbe multiple choice question , it will handle user input and display impressive results.
Preview Of Quiz App Using ReactJS
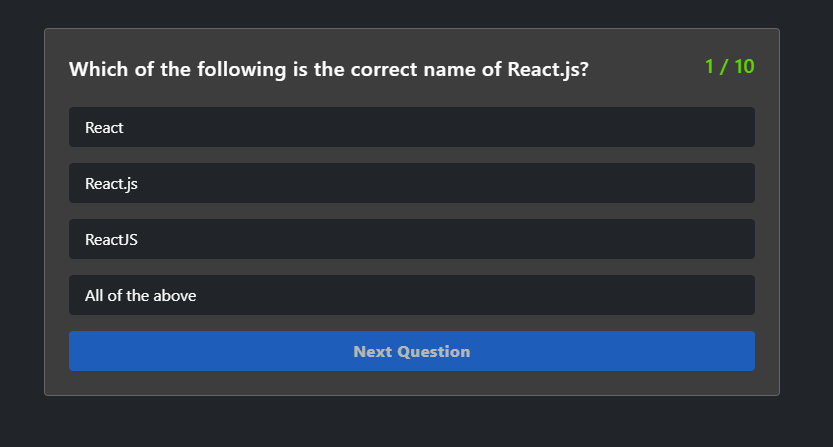
Prerequisite
You must have basic knowledge of the below mentioned tool and technology:-
- HTML
- CSS
- JavaScript
- VsCode Editor
50+ Interesting React JS Project ideas With Source Code from Beginner and Intermediate-level
Set Up the Project
Before directly jump intot he code and start building the project ypu must need to setup the reactjs in your File Folder.
Open your terminal and run the following command:
npx create-react-app quiz-app
This command will create a new React.js project named “quiz-app.”
Create this project in desired folder sturcture and then open this in ypur Visual Studio Code.
ReactJS Code for Quiz App
Below is the folder structure For Quiz-App should look like
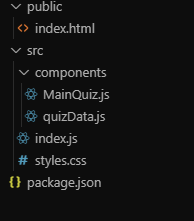
MainQuiz.js
Create a MainQuiz.js File and paste the following code:-
import React from "react";
import { quizData } from "./quizData";
class MainQuiz extends React.Component {
state = {
currentQuestion: 0,
myAnswer: null,
options: [],
score: 0,
disabled: true,
isEnd: false
};
loadQuizData = () => {
// console.log(quizData[0].question)
this.setState(() => {
return {
questions: quizData[this.state.currentQuestion].question,
answer: quizData[this.state.currentQuestion].answer,
options: quizData[this.state.currentQuestion].options
};
});
};
componentDidMount() {
this.loadQuizData();
}
nextQuestionHandler = () => {
// console.log('test')
const { myAnswer, answer, score } = this.state;
if (myAnswer === answer) {
this.setState({
score: score + 1
});
}
this.setState({
currentQuestion: this.state.currentQuestion + 1
});
console.log(this.state.currentQuestion);
};
componentDidUpdate(prevProps, prevState) {
if (this.state.currentQuestion !== prevState.currentQuestion) {
this.setState(() => {
return {
disabled: true,
questions: quizData[this.state.currentQuestion].question,
options: quizData[this.state.currentQuestion].options,
answer: quizData[this.state.currentQuestion].answer
};
});
}
}
//check answer
checkAnswer = answer => {
this.setState({ myAnswer: answer, disabled: false });
};
finishHandler = () => {
if (this.state.currentQuestion === quizData.length - 1) {
this.setState({
isEnd: true
});
}
if (this.state.myAnswer === this.state.answer) {
this.setState({
score: this.state.score + 1
});
}
};
render() {
const { options, myAnswer, currentQuestion, isEnd } = this.state;
if (isEnd) {
return (
<div className="result">
<h3>Game Over your Final score is {this.state.score} points </h3>
<div>
The correct answer's for the questions was
<ul>
{quizData.map((item, index) => (
<li className="ui floating message options" key={index}>
{item.answer}
</li>
))}
</ul>
</div>
</div>
);
} else {
return (
<div className="App">
<h1>{this.state.questions} </h1>
<span>{`Questions ${currentQuestion} out of ${quizData.length -
1} remaining `}</span>
{options.map(option => (
<p
key={option.id}
className={`ui floating message options
${myAnswer === option ? "selected" : null}
`}
onClick={() => this.checkAnswer(option)}
>
{option}
</p>
))}
{currentQuestion < quizData.length - 1 && (
<button
className="ui inverted button"
disabled={this.state.disabled}
onClick={this.nextQuestionHandler}
>
Next
</button>
)}
{/* //adding a finish button */}
{currentQuestion === quizData.length - 1 && (
<button className="ui inverted button" onClick={this.finishHandler}>
Finish
</button>
)}
</div>
);
}
}
}
export default MainQuiz;
quizData.js
Create another Fiele name as quizData.js in the Component folder and add the following code:-
This File will be used to define the Question and answers for the quiz.
export const quizData = [ { id: 0, question: ` What is the Capital Of India ?`, options: [`New Delhi`, `Mumbai`, `Kolkatta`], answer: `New Delhi` }, { id: 1, question: `Who is the CEO of Tesla Motors?`, options: [`Bill Gates`, `Steve Jobs`, `Elon Musk`], answer: `Elon Musk` }, { id: 3, question: `Name World's Richest Man?`, options: [`Jeff Bezo`, `Bill Gates`, `Mark Zuckerberg`], answer: `Jeff Bezo` }, { id: 4, question: `World's Longest River?`, options: [`River Nile`, `River Amazon`, `River Godavari`], answer: `River Nile` } ];
index.js
Create and file inseide the src folder name as index.js and paste the following code:-
import React from "react"; import ReactDOM from "react-dom"; import "./styles.css"; import MainQuiz from "./components/MainQuiz"; function App() { return ( <div className="App"> <MainQuiz /> </div> ); } const rootElement = document.getElementById("root"); ReactDOM.render(<App />, rootElement);
Styling Our Quiz-App
Now let’s style the our App. Add the style.css file inside the src folder and paste the following code:-
.App { font-family: sans-serif; text-align: center; color: #f1f2f6; } body { font-size: 1.2rem; font-family: -apple-system, BlinkMacSystemFont, "Segoe UI", Roboto, Oxygen, Ubuntu, Cantarell, "Open Sans", "Helvetica Neue", sans-serif; background: #bdc3c7; /* fallback for old browsers */ background: -webkit-linear-gradient( to right, #2c3e50, #bdc3c7 ); /* Chrome 10-25, Safari 5.1-6 */ background: linear-gradient(to right, #2c3e50, #bdc3c7); } .result { margin-top: 20px; } .options { cursor: pointer; border: 2px solid black; padding: 10px; } .selected { background: linear-gradient(to right, #74ebd5, #acb6e5) !important; } ul { list-style-type: none; }
Dependencies
Your package.json file should be look like this
{ "name": "react-quiz-app", "version": "1.0.0", "description": "", "keywords": [], "main": "src/index.js", "dependencies": { "react": "16.8.6", "react-dom": "16.8.6", "react-scripts": "3.0.1" }, "devDependencies": { "typescript": "3.3.3" }, "scripts": { "start": "react-scripts start", "build": "react-scripts build", "test": "react-scripts test --env=jsdom", "eject": "react-scripts eject" }, "browserslist": [ ">0.2%", "not dead", "not ie <= 11", "not op_mini all" ] }
Final Preview of our Quiz-App Using ReactJS
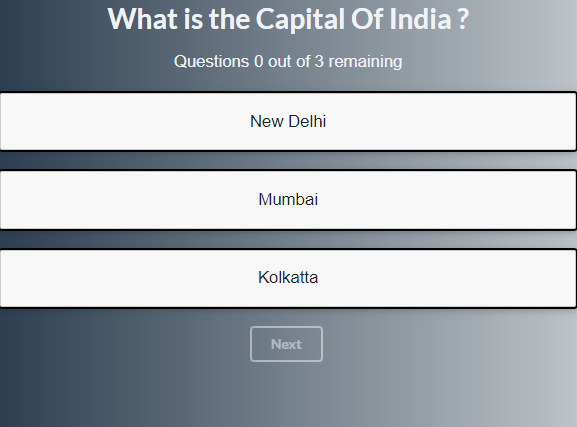
Conclusion
Congratulation , You have completed your Mini Reactjs Project and further you can enhace this as per your preferences. You can deploy it on the Github, Vercel or netfliy platform and make it live for your freinds. Add this into ypur resumes after adding or enhancing more functionality like timer, stopwatch, profile , saving progress and many more.
I hope you liked this Tutorial and must have learned Something new. If ypu have any questions Regarding this feel free to drop your comments below and contact with our team on Intagram @Codewithrandom .
Code Credit:- NinjaAniket
HAPPY CODING!!