Create Incremental and Decremental counter using HTML, CSS and JavaScript
Hey coders, in this article you will learn how to Create Incremental and Decremental counter using HTML, CSS, and JavaScript – Fully responsive.
In a counter application, when you click on the plus(+) button, the counter increases the count by one and for the decrement of the count, you click on the minus(-) button. We will also create a reset button to reset the count value.
To create this project, we will be creating three files (HTML file, a CSS file, and JavaScript File). The HTML file to create the basic structure, CSS file to add styling t our project and javascript to provide logic to our counter. Let us now start creating the counter.
Step 1: Adding basic HTML
Creating basic structure of Counter using HTML – Hypertext Markup Language. Here is the code for you to directly use it. The code will be explained below for you to understand how to create the Counter.
<html> <head> <link rel="stylesheet" href="counter.css"> </head> <body> <div id="main"> <div id="displayCounter"></div><br> <button id="increment"> + </button> <button id="decrement"> - </button> <button id="reset">Reset</button><br><br> </div> <script src="counter.js"></script> </body> </html>
Let us now start understanding html for this project:
- Inside the head section of the html, we will link the CSS file. You can name the stylesheet according to you.
- Then we will start creating the structure inside the body tag. We will create a division using the div tag and associate an id to it, id=”main”.
- Inside the main div we will nest another div with id=”displayCounter”.
- Then we will create three buttons using the button tag for increment, decrement and reset of the counter value.
- Linking the javascript for the logic by using the script tag.
Weather App Using Html,Css And JavaScript
OUTPUT:
Step 2:- Adding JavaScript to provide logic behind the buttons
Here is the code, you can copy it to directly use it in your IDE for this project.
var productCounter={ count:0, incrementCounter:function(){ if(this.count<10){ return this.count = this.count + 1; }else{ alert("maximum limit reached, you can buy only 10 items"); return this.count; } }, decrementCounter:function(){ if (this.count>0){ return this.count = this.count - 1; } else { return this.count=0; } }, resetCounter:function(){ return this.count=0; } }; var displayCout = document.getElementById('displayCounter'); displayCout.innerHTML=0; document.getElementById('increment').onclick=function(){ displayCout.innerHTML=productCounter.incrementCounter(); } document.getElementById('decrement').onclick=function(){ displayCout.innerHTML = productCounter.decrementCounter(); } document.getElementById('reset').onclick=function(){ displayCout.innerHTML = productCounter.resetCounter(); }
Let us understand the Javascript code:-
- Create a variable called productCounter and set a count starting value to 0. We will create a funtion for the increment called incrementCounter and give functionality to it. The max count value for this project is 10 but you can set that value according to your requirement. If the count reaches 10 and we try to increment it then we want to get an alert,alert(“maximum limit reached, you can buy only 10 items”);
50+ HTML, CSS & JavaScript Projects With Source Code
Now we will create a decrement function called
decrementCounter and add functionality to subtract the count by 1 when clicked on the minus(-) button.- To reset the value of the counter, we have created a reset button and now we will give functionality to it by creating a reset function called resetCounter which will return 0 when clicked on the button.
After setting the button functionalities for increment, decrement and reset, we will associate these functions to the buttons. Doing this by creating a variable called displayCout which will return the element by id –
var displayCout = document.getElementById(‘displayCounter’);- Setting the value of innerHTML of displayCout variable as 0, as we want to begin the count from 0.
- The method of returning the element by id with the onclick event. The onclick event occurs when the user clicks on an HTML element.document.getElementById(‘increment’).onclick=function(){displayCout.innerHTML=productCounter.incrementCounter();}
- Similary we will associate the functions with onlick event for the decrement and reset as well.
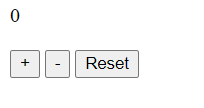
Step 3: Add CSS to style the basic structure of the Counter
#increment, #decrement, #reset{ padding:10px 15px; font-size:18px; background: #56ccf2; background: -webkit-linear-gradient(to right, #56ccf2, #2f80ed); background: linear-gradient(to right, #56ccf2, #2f80ed); color:#f1f1f1; border:none; margin:5px; } #main{ margin : 0 auto; text-align:center; padding:5% 10%; } #displayCounter{ font-size:100px; font-family: impact; }
Let us understand the CSS code:-
- We will target the buttons with their ids- id=”increment”, id=”decrement”, id=”reset” using the # selector and style the following properties with the values – padding:10px 15px; font-size:18px; background: #56ccf2; background: -webkit-linear-gradient(to right, #56ccf2, #2f80ed); background: linear-gradient(to right, #56ccf2, #2f80ed); color:#f1f1f1; border:none; margin:5px;
- For the main div with id=”main”, targeting it by # selector, we will align the html elements on the webpage at the center using the declaration text-align:center; and add margin: 0 auto; along with padding:5% 10%; .
- At last we will style the html element with id=”displayCounter”, which will display the count with the following properties – font-size:100px; font-family: impact; .
Ecommerce Website Using HTML, CSS, & JavaScript (Source Code)
OUTPUT: With HTML, CSS and Javascript combined-
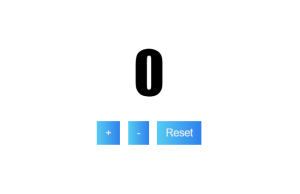
I hope that this article was helpful and you understood the whole project. Now let’s take a look at the Live Preview of the Counter.
Live Preview On Codepen Incremental and Decremental counter
Below is a Codepen preview Of Counter Application Using the JavaScript.
Conclusion
We have successfully created a Counter using HTML, CSS & JavaScript. You can use this project directly by copying it into your IDE. We hope you understood the project, If you have any doubts feel free to comment!!.
I hope you liked the article and got to learn something. Please do checkout our other Articles for free source code.
Happy exploring and learning !!
Follow: codeWithRandom
Code by: Akash Web – Jackie
Written by: Cheshta Vohra
HAPPY CODING!!!