How to Create Palindrome Checker Using HTML , CSS & JavaScript
This tutorial will teach you how to develop a palindrome checker in HTML, CSS, and JavaScript. To make this palindrome checker in JavaScript.
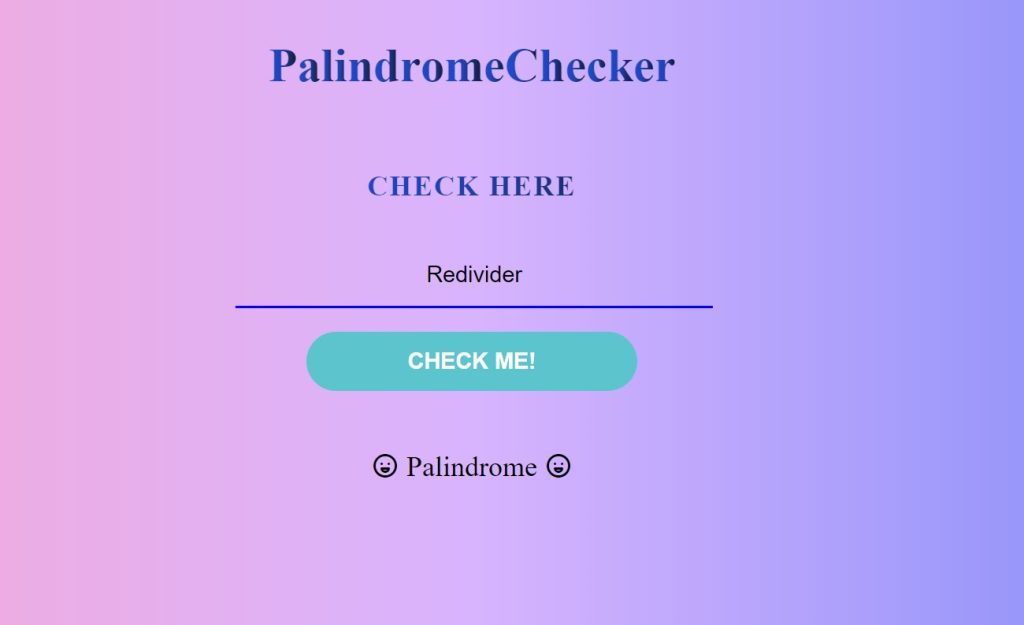
A palindrome is a word, phrase, or sentence that reads the same when read backward as it does when read forward. A palindrome occurs when the numbers, strings, or characters are reversed and produce the same outcome as the original numbers or letters. For instance, Redivider, 123454321, and so on. Let’s say we have a word: redivider. When we read the word “redivider” from the front and back ends, we get the same string. As a result, we can call the string or number a palindrome.
Palindrome Algorithm:
- Get the user’s strings or numbers.
- Consider a temporary variable that stores the numbers.
- Reverse the given number.
- Compare the original and inverted numbers.
- A palindrome is a number or string that has the same temporary and original numbers.
- If the given string or number is not a palindrome, it is not a palindrome.
I hope you have a general idea of what the project entails. In our article, we will go over this project step by step.
Step1: Adding HTML Markup
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8" /> <meta http-equiv="X-UA-Compatible" content="IE=edge" /> <meta name="viewport" content="width=device-width, initial-scale=1.0" /> <link rel="stylesheet" href="style.css" /> <link rel="stylesheet" href="https://unicons.iconscout.com/release/v4.0.0/css/line.css" /> <title>Palindrome Checker</title> </head> <body> <div class="container"> <h1 class="title">Palindrome Checker</h1> <!-- ------- BOX FOR THE PALINDROME --------------- --> <div class="palindrome__box"> <h2 class="box__title">Check Here</h2> <div class="input__box"> <input type="text" class="text__input" placeholder="Put your word here!" /> <button class="submit__button" type="submit">Check Me!</button> <div class="results"></div> </div> </div> </div> <script src="script.js"></script> </body> </html>
We’ll start by adding the structure of the project, but first we’ll need to include some items inside the link, such as the fact that we’ve utilised numerous CSS and javascript files, which we’ll need to connect up inside our HTML file.We also utilised some icons in our palindrome checker app, so we need to include some icon links inside the head part of our HTML to include those symbols in our project.
Style and icon links in head section <link rel="stylesheet" href="style.css" /> <link rel="stylesheet" href="https://unicons.iconscout.com/release/v4.0.0/css/line.css" /> Javascript link in body section <script src="script.js"></script>
Adding the Structure for our Palindrome Checker app:
- Using the <div> tag we will create a container for our palindrome checker app.
- We’ll give our palindrome project a heading now by using the <h1> tag.
- We’ll use the <div> tag with the class “palindrome box” to create a box for the palindrome.
- Now, inside our palindrome checker, we’ll add a header “Check here” with the <h2> tag.
- Now we will create an input box which will take input from the user.
- We’ll make a button to check the solution using the button tag.
- We will also add a div tag to help us determine whether the string or number supplied is a palindrome or not.
Ecommerce Website Using Html Css And Javascript Source Code
We will just use some simple html tags to construct the structure for our palindrome checker, and we will add styling to our wrapper class using the CSS concepts.
Output:
Step2: Adding CSS Code
In your stylesheet, copy and paste the CSS code provided below. To add styling to our browser, we will use the class selector.
body { font-size: 62.5%; background: linear-gradient( to right, rgb(249, 168, 212), rgb(216, 180, 254), rgb(129, 140, 248) ); } .container { position: absolute; top: 40%; left: 50%; width: 400px; height: 400px; text-align: center; transform: translate(-50%, -50%); } .palindrome__box { margin: 50px auto; width: 100%; height: 100%; } .box__title { padding: 15px; font-weight: bold; font-size: 1.5rem; letter-spacing: 1.5px; text-align: center; text-transform: uppercase; background: linear-gradient( to right, rgb(29, 78, 216), rgb(30, 64, 175), rgb(17, 24, 39) ); color: transparent; background-clip: text; -webkit-background-clip: text; } .input__box { width: 100%; height: 50px; } .text__input { width: 70%; border: none; background: none; text-align: center; height: 100%; font-size: 1.2rem; margin-bottom: 20px; border-bottom: 2px solid blue; transition: all 0.4s ease-in; } .text__input:focus { width: 100%; outline: none; } .submit__button { width: 70%; height: 50px; border: none; color: #fff; cursor: pointer; font-size: 1.2rem; text-transform: uppercase; font-weight: 700; border-radius: 100px; animation: background 2s linear infinite; transition: all 2s ease-in; } .submit__button:hover { background: linear-gradient( to right, rgb(110, 231, 183), rgb(59, 130, 246), rgb(147, 51, 234) ); animation: none; } .results { margin: 20px auto; font-size: 1.5rem; padding-left: 10px; overflow-wrap: break-word; padding: 30px 0; } span { font-weight: bold; font-size: 2.5rem; display: inline-block; transition: 1s ease-in; background: linear-gradient( to right, rgb(29, 78, 216), rgb(30, 64, 175), rgb(17, 24, 39) ); color: transparent; background-clip: text; -webkit-background-clip: text; } span.fade { animation: title 0.4s ease-in 1; } @keyframes title { 0% { transform: scale(1); } 50% { transform: scale(1.5); } 100% { transform: scale(1); } } @keyframes background { 0% { background: rgb(110, 231, 183); } 50% { background: rgb(59, 130, 246); } 100% { background: rgb(147, 51, 234); } }
Step1: Using the body tag selector, we will set the font size of our body to 62.5%, and we will add a linear gradient colour to our body using the background property. In the gradient, we will use a combination of light purple, pink, and blue.
body { font-size: 62.5%; background: linear-gradient( to right, rgb(249, 168, 212), rgb(216, 180, 254), rgb(129, 140, 248) ); }
Step2: Using the class selector, we will now style our container and palindrome box. Using the top and left attributes, we will leave 40% space from the top and 50% space from the left. The height and width of our container are set to 400 pixels, respectively. We will centre the text by using the text-align attribute.
Using the class selector for our palindrome box, we will now add a 50px margin to our palindrome box, with the height and width set to 100%.
.container { position: absolute; top: 40%; left: 50%; width: 400px; height: 400px; text-align: center; transform: translate(-50%, -50%); } .palindrome__box { margin: 50px auto; width: 100%; height: 100%; }
Step3: Using the class selector, we will now style our heading and other elements. We will add a padding of 15px to our box title using the class selector (.box title), and we will set the font-weight to “bold” using the font-weight property.
5+ HTML CSS Projects With Source Code
We will use the.input box to set the width to 100% and the height to 50px.
.box__title { padding: 15px; font-weight: bold; font-size: 1.5rem; letter-spacing: 1.5px; text-align: center; text-transform: uppercase; background: linear-gradient( to right, rgb(29, 78, 216), rgb(30, 64, 175), rgb(17, 24, 39) ); color: transparent; background-clip: text; -webkit-background-clip: text; } .input__box { width: 100%; height: 50px; } .text__input { width: 70%; border: none; background: none; text-align: center; height: 100%; font-size: 1.2rem; margin-bottom: 20px; border-bottom: 2px solid blue; transition: all 0.4s ease-in; } .text__input:focus { width: 100%; outline: none;
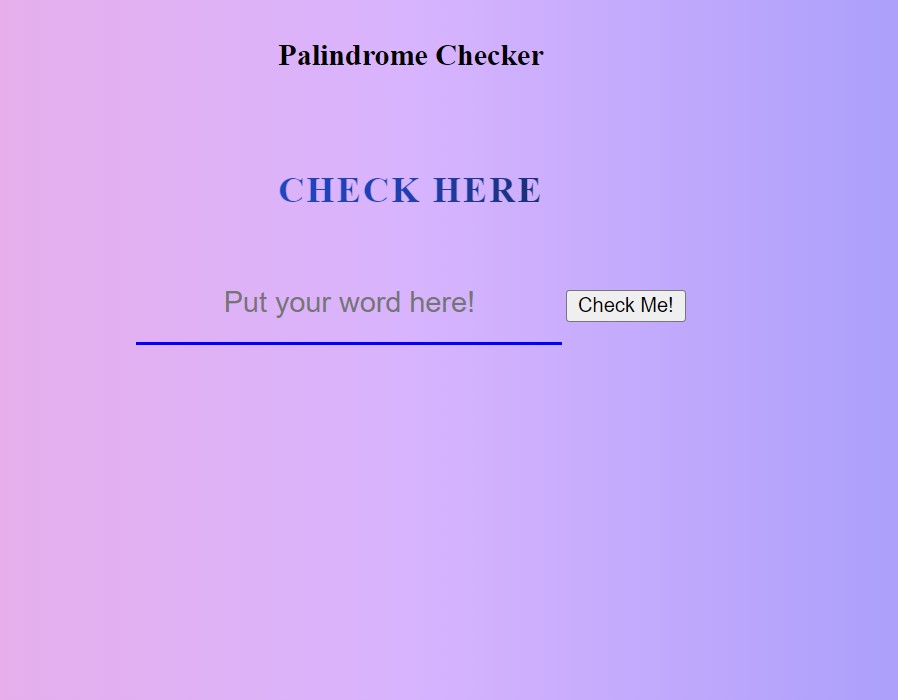
Step5:Now we’ll style the button and add some animation effects to our palindrome checker. We’ll use the (.submit button) to set the width to 70% and the height to 50px. We’ll set the font size to 1.2 rem using the font-size property. We will change the text to uppercase using the text-transform property. We’ll also include a hover effect, in which the button’s background colour changes as the user hovers over it.
We’ll use the keyframe to add animation to the background and title of our palindrome checker.
ADVERTISEMENT
Step3: JavaScript Code
Finally, create a JavaScript file called script.js and paste the following codes into it. Remember to save the file with the.js extension.
ADVERTISEMENT
const title = document.querySelector(".title"); const titleContent = title.textContent; const input = document.querySelector(".text__input"); const submitButton = document.querySelector(".submit__button"); let resultBox = document.querySelector(".results"); // trophy icon let winIcon = `<i class="uil uil-grin"></i>`; let loseIcon = `<i class="uil uil-sad-cry"></i>`; // Making the animation happen as soon as the page was loaded window.onload = function () { // We should split up the word / the result is always an array const splitedText = titleContent.split(""); title.textContent = ""; // Creating a for loop for every charachter to changed into a span for (let i = 0; i < splitedText.length; i++) { title.innerHTML += `<span>${splitedText[i]}</span>`; } let char = 0; let timer = setInterval(onClick, 100); function onClick() { const span = title.querySelectorAll("span")[char]; span.classList.add("fade"); // increasing it to be happended for every one of them char++; //we need to stop it if (char === splitedText.length) { complete(); return; } } function complete() { clearInterval(timer); timer = null; } }; // checking the users word submitButton.addEventListener("click", palindromeChecker); // palindrome checker function palindromeChecker(word) { word = input.value.toLowerCase(); // console.log(word); let wordSplited = word.split(""); // console.log(wordSplited); let reversedWord = wordSplited.reverse(); //joining the reversed word let joinedWord = reversedWord.join(""); // console.log(reversedWord.join("")); // Setting the conditions / 1.Not empty 2. Exactly the same with it's reversed if (word == joinedWord && word != "") { // console.log('It is a palindrppme'); // Adding the result to the box resultBox.innerHTML = `${winIcon} Palindrome ${winIcon}`; input.value = ""; } else if (word == "") { resultBox.innerHTML = "Enter a Word!"; } else { // console.log('It is not a palindrome'); // Adding the result to the box resultBox.innerHTML = `${loseIcon} Not A Palindrome ${loseIcon}`; input.value = ""; } }
- We will first select the html elements using the document.queryselector() method
- Using the let keyword, we will now create two variables and store the icons for winning and losing inside them.
- We’ll make the animation with the onload method, and we’ll split up the word with the split() method; the result is always an array.
- Now we’ll write a onclick method, add a class, and increase the char value by one.
- Now we’ll add an event listener to our palindrome checker and see if the string we gave is a palindrome. To accomplish this, we will construct a variable and store our string into it. The string will then be reversed. If the reverse string has the same value as the original string, it is a palindrome; otherwise, it is not a palindrome. The result will then be displayed to the user using HTML.
50+ Html ,Css & Javascript Projects With Source Code
ADVERTISEMENT
Now we’ve completed our palindrome checker app using HTML , CSS & javascript. I hope you understood the whole project. Let’s take a look at our Live Preview.
ADVERTISEMENT
Output:
ADVERTISEMENT
Live Preview Of Palindrome checker using HTML , CSS & Javascript
Now We have Successfully created our palindrome checker using HTML , CSS & javascript. You can use this project directly by copying into your IDE. WE hope you understood the project , If you any doubt feel free to comment!!
If you find out this Blog helpful, then make sure to search code with random on google for Front End Projects with Source codes and make sure to Follow the Code with Random Instagram page.